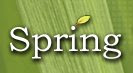
The Spring Framework (or Spring for short) is an open source application framework for the Java platform. The first version was written by Rod Johnson, who first released it with the publication of his book Expert One-on-One J2EE Design and Development (Wrox Press, October 2002). A port is available for the .NET Framework.[1]. The Spring 1.2.6 framework won a Jolt productivity award in 2006 [2]
Although the Spring Framework does not enforce any specific programming model, it has become popular in the Java community as an alternative, replacement, or even addition to the Enterprise JavaBean (EJB) model. By design, the framework offers a lot of freedom to Java developers yet provides well documented and easy-to-use solutions for common practices in the industry.
While the core features of the Spring Framework can be used by any Java application, there are many extensions and improvements for building web-based applications on top of the Java Enterprise platform. Spring is popular because of this, and is recognized by vendors as a strategically important framework. [3]
The framework was first released under the Apache 2.0 license in June 2003. The first milestone release, 1.0, was released in March 2004, with further milestone releases in September 2004 and March 2005. Current version is 2.5.5.
Introduction
The Spring Framework provides solutions to many technical challenges faced by Java developers and organizations wanting to create applications based on the Java platform. Because of the size and complexity of the functionality offered, it can be hard to distinguish the major building blocks from which the framework is composed.[neutrality disputed] The Spring Framework is not exclusively linked to the Java Enterprise platform, although its far-reaching integration in this area is an important reason for its popularity.
The Spring Framework is probably best known for offering features required to create complex business applications effectively outside of the programming models which have been dominant historically in the industry.[neutrality disputed] Next to that, it is also credited for introducing previously unfamiliar functionalities into today's mainstream development practices, even beyond the Java platform.
This amounts to a framework which offers a consistent model and makes it applicable to most application types created on top of the Java platform.
Modules
The Spring Framework can be considered as a collection of smaller frameworks. Most of these frameworks are designed to work independently of each other yet provide better functionalities when used together. These frameworks are divided along the building blocks of typical complex applications:
- Inversion of Control container: configuration of application components and lifecycle management of Java objects.
- Aspect-oriented programming framework: working with functionalities which cannot be implemented with Java's object-oriented programming capabilities without making sacrifices.
- Data access framework: working with relational database management systems on the Java platform using JDBC and Object-relational mapping tools providing solutions to technical challenges which are reusable in a multitude of Java-based environments.
- Transaction management framework: harmonization of various transaction management API's and configurative transaction management orchestration for Java objects.
- Model-view-controller framework: HTTP and Servlet based framework providing many hooks for extension and customization.
- Remote Access framework: configurative RPC-style export and import of Java objects over computer networks supporting RMI, CORBA and HTTP-based protocols including web services (SOAP).
- Authentication and authorization framework: configurative orchestration of authentication and authorization processes supporting many popular and industry-standard standards, protocols, tools and practices via the Spring Security sub-project (formerly Acegi).
- Remote Management framework: configurative exposure and management of Java objects for local or remote configuration via JMX.
- Messaging framework: configurative registration of message listener objects for transparent message consumption from message queues via JMS, improvement of message sending over standard JMS API's.
- Testing framework: support classes for writing unit tests and integration tests.
Inversion of Control container
Central in the Spring Framework is its Inversion of Control container, which provides a consistent means of configuring and managing Java objects using callbacks. This container is also known as BeanFactory, ApplicationContext, or Core container.
The container has many responsibilities and extension points which can all be considered as forms of Inversion of Control, hence its name. Examples are: creating objects, configuring objects, calling initialization methods, and passing objects to registered callback objects. Many of the functionalities of the container together form the object lifecycle, which is one of the most important features it provides.
Objects created by the container are also called Managed Objects or Beans. Typically the container is configured by loading XML files which contain Bean definitions. These provide all the information required to create objects. Once objects are created and configured without raising error conditions, they become available for use.
Objects can be obtained by means of Dependency lookup or Dependency injection. Dependency lookup is a pattern where a caller asks the container object for an object with a specific name or of a specific type. Dependency injection is a pattern where the container passes objects by name to other objects, via either constructors, properties, or factory methods.
In many cases it's not necessary to use the container when using other parts of the Spring Framework, although using it will likely make an application easier to configure and customize. The Spring container provides a consistent mechanism to configure applications and integrates with almost all Java environments, from small-scale applications to large enterprise applications[citation needed].
The container can be turned into a partially-compliant EJB3 container by means of the Pitchfork project. The Spring Framework is criticized by some as not being standards compliant. However, SpringSource doesn't see EJB3 compliance as a major goal, and claims that the Spring Framework and the container allow for more powerful programming models.[4]
Aspect-oriented programming framework
The Spring Framework has its own AOP framework which modularizes cross-cutting concerns in aspects. The motivation for creating a separate AOP framework comes from the belief that it would be possible to provide basic AOP features without too much complexity in either design, implementation, or configuration. The Spring AOP framework also takes full advantage of the Spring Container.
The Spring AOP framework is interception based, and is configured at runtime. This removes the need for a compilation step or load-time weaving. On the other hand, interception only allows for public or protected method execution on existing objects at a join point.
Compared to the AspectJ framework, Spring AOP is less powerful but also less complicated. Spring 1.2 includes support to configure AspectJ aspects in the container. Spring 2.0 has more integration with AspectJ; for example, the pointcut language is reused.
Spring AOP has been designed to make it able to work with cross-cutting concerns inside the Spring Framework. Any object which is created and configured by the container can be enriched using Spring AOP.
The Spring Framework uses Spring AOP internally for transaction management, security, remote access, and JMX.
Since version 2.0 of the framework, Spring provides two approaches to the AOP configuration:
- schema-based approach
- @AspectJ-based annotation style
The Spring team decided not to introduce new AOP-related terminology; therefore, in the Spring reference documentation and API, terms such as aspect, join point, advice, pointcut, introduction, target object (advised object), AOP proxy, and weaving all have the same meanings as in most other AOP frameworks (particularly AspectJ).
Data access framework
Spring's data access framework addresses common difficulties developers face when working with databases in applications. Support is provided for all popular data access frameworks in Java: JDBC[1], iBatis[2], Hibernate[3], JDO[4], JPA[5], Oracle TopLink, Apache OJB[6], and Cayenne[7], among others.
For all of these supported frameworks, Spring provides these features:
- Resource management - automatically acquiring and releasing database resources
- Exception handling - translating data access related exception to a Spring data access hierarchy
- Transaction participation - transparent participation in ongoing transactions
- Resource unwrapping - retrieving database objects from connection pool wrappers
- Abstraction for BLOB and CLOB handling
All these features become available when using Template classes provided by Spring for each supported framework. Critics say these Template classes are intrusive and offer no advantage over using (for example) the Hibernate API directly.[8] In response, the Spring developers have made it possible to use the Hibernate and JPA APIs directly. This however requires transparent transaction management, as application code no longer assumes the responsibility to obtain and close database resources, and does not support exception translation.
Together with Spring's transaction management, its data access framework offers a flexible abstraction for working with data access frameworks. The Spring Framework doesn't offer a common data access API; instead, the full power of the supported APIs is kept intact. The Spring Framework is the only framework available in Java which offers managed data access environments outside of an application server or container.[citation needed]
While using Spring for transaction management with Hibernate, following beans may be required to be configured
- DataSource like com.mchange.v2.c3p0.ComboPooledDataSource or org.apache.commons.dbcp.BasicDataSource
- SessionFactory like org.springframework.orm.hibernate3.LocalSessionFactoryBean
- HibernateProperties like org.springframework.beans.factory.config.PropertiesFactoryBean
- TransactionManager like org.springframework.orm.hibernate3.HibernateTransactionManager
Other configurations
- AOP configuration of cutting points using
- Transaction semantics of AOP advice using
Transaction management framework
Spring's transaction management framework brings an abstraction mechanism to the Java platform. Its abstraction is capable of:
- working with local and global transactions (local transaction does not require an application server)
- working with nested transactions
- working with transaction safepoints
- working in almost all environments of the Java platform
In comparison, JTA only supports nested transactions and global transactions, and requires an application server (and in some cases also deployment of applications in an application server).
The Spring Framework ships a PlatformTransactionManager for a number of transaction management strategies:
- Transactions managed on a JDBC Connection
- Transactions managed on Object-relational mapping Units of Work
- Transactions managed via the JTA TransactionManager and UserTransaction
- Transactions managed on other resources, like object databases
Next to this abstraction mechanism the framework also provides two ways of adding transaction management to applications:
- Programmatically, by using Spring's TransactionTemplate
- Configuratively, by using metadata like XML or Java 5 annotations
Together with Spring's data access framework — which integrates the transaction management framework — it is possible to set up a transactional system through configuration without having to rely on JTA or EJB. The transactional framework also integrates with messaging and caching engines.
Model-view-controller framework
The Spring Framework features its own MVC framework, which wasn't originally planned. The Spring developers decided to write their own web framework as a reaction to what they perceived as the poor design of the popular Jakarta Struts web framework[9], as well as deficiencies in other available frameworks. In particular, they felt there was insufficient separation between the presentation and request handling layers, and between the request handling layer and the model.[5]
Like Struts, Spring MVC is a request-based framework. The framework defines strategy interfaces for all of the responsibilities which must be handled by a modern request-based framework. The responsibility of each interface is sufficiently simple and clear that it's easy for Spring MVC users to write their own implementations if they choose to[neutrality disputed]. All interfaces are tightly coupled to the Servlet API to offer the full power of this API. This tight coupling to the Servlet API is seen by some as a failure on the part of the Spring developers to offer a high-level abstraction for web-based applications[citation needed]. However, this coupling makes sure that the features of the Servlet API remain available to developers while offering a high abstraction framework to ease working with said API.
The DispatcherServlet class is the front controller [10] of the framework and is responsible for delegating control to the various interfaces during the execution phases of an HTTP request.
The most important interfaces defined by Spring MVC, and their responsibilities, are listed below:
- HandlerMapping: selecting objects which handle incoming requests (handlers) based on any attribute or condition internal or external to those requests
- HandlerAdapter: execution of objects which handle incoming requests
- Controller: comes between Model and View to manage incoming requests and redirect to proper response.
- View: responsible for returning a response to the client
- ViewResolver: selecting a View based on a logical name for the view (use is not strictly required)
- HandlerInterceptor: interception of incoming requests comparable but not equal to Servlet filters (use is optional and not controlled by DispatcherServlet).
- LocaleResolver: resolving and optionally saving of the locale of an individual user
- MultipartResolver: facilitate working with file uploads by wrapping incoming requests
Each strategy interface above has an important responsibility in the overall framework. The abstractions offered by these interfaces are sufficiently powerful to allow for a wide set of variations in their implementations. Spring MVC ships with implementations of all these interfaces and together offers a powerful feature set on top of the Servlet API[neutrality disputed]. However, developers and vendors are free to write other implementations. Spring MVC uses the Java java.util.Map
interface as a data-oriented abstraction for the Model where keys are expected to be string values.
The ease of testing the implementations of these interfaces is one important advantage of the high level of abstraction offered by Spring MVC. DispatcherServlet is tightly coupled to the Spring Inversion of Control container for configuring the web layers of applications. However, applications can use other parts of the Spring Framework—including the container—and choose not to use Spring MVC.
Because Spring MVC uses the Spring container for configuration and assembly, web-based applications can take full advantage of the Inversion of Control features offered by the container.
Remote access framework
Spring's Remote Access framework is an abstraction for working with various RPC-based technologies available on the Java platform both for client connectivity and exporting objects on servers. The most important feature offered by this framework is to ease configuration and usage of these technologies as much as possible by combining Inversion of Control and AOP.
The framework also provides fault-recovery (automatic reconnection after connection failure) and some optimizations for client-side use of EJB remote stateless session beans.
Spring provides support for these protocols and products out of the box:
- HTTP-based protocols
- Hessian: binary serialization protocol, open-sourced and maintained by Corba-based protocols
- RMI (1): method invocations using RMI infrastructure yet specific to Spring
- RMI (2): method invocations using RMI interfaces complying with regular RMI usage
- RMI-IIOP (Corba): method invocations using RMI-IIOP/Corba
- Enterprise JavaBean client integration
- Local EJB stateless session bean connectivity: connecting to local stateless session beans
- Remote EJB stateless session bean connectivity: connecting to remote stateless session beans
- SOAP
- Integration with the Apache Axis web services framework
The XFire SOAP framework provides integration with the Spring Framework for RPC-style exporting of object on the server side.
Both client and server setup for all RPC-style protocols and products supported by the Spring Remote access framework (except for the Apache Axis support) is configured in the Spring Core container.
There is alternative open-source implementation (Cluster4Spring) of a remoting subsystem included into Spring Framework which is intended to support various schemes of remoting (1-1, 1-many, dynamic services discovering).
References
- ^ Spring.NET - Application Framework
- ^ Jolt winners 2006
- ^ http://ostatic.com/67409-software-opensource/spring
- ^ "Pitchfork FAQ". Retrieved on 2006-06-06.
- ^ Johnson, Expert One-on-One J2EE Design.., Ch. 12. et al.
- Johnson, Rod; Jürgen Höller, Alef Arendsen, Thomas Risberg, and Colin Sampaleanu (2005). Professional Java Development with the Spring Framework. Wiley. ISBN 0-7645-7483-3.
- Harrop, Rob; Jan Machahek (2005). Pro Spring. APress. ISBN 1-59059-461-4.
- Johnson, Rod; Jürgen Höller (2004). J2EE Development without EJB. Wiley. ISBN 0-7645-5831-5.
- Johnson, Rod (2002). Expert One-on-one J2EE Design and Development. Wiley. ISBN 0-7645-4385-7.
- Walls, Craig; Ryan Breidenbach (2005). Spring in Action. Manning. ISBN 1-9323-9435-4.
- Wolff, Eberhard (2006). Spring - Framework für die Java Entwicklung. dpunkt. ISBN 3-89864-365-4.
No comments:
Post a Comment